Using Mockito with JUnit 5
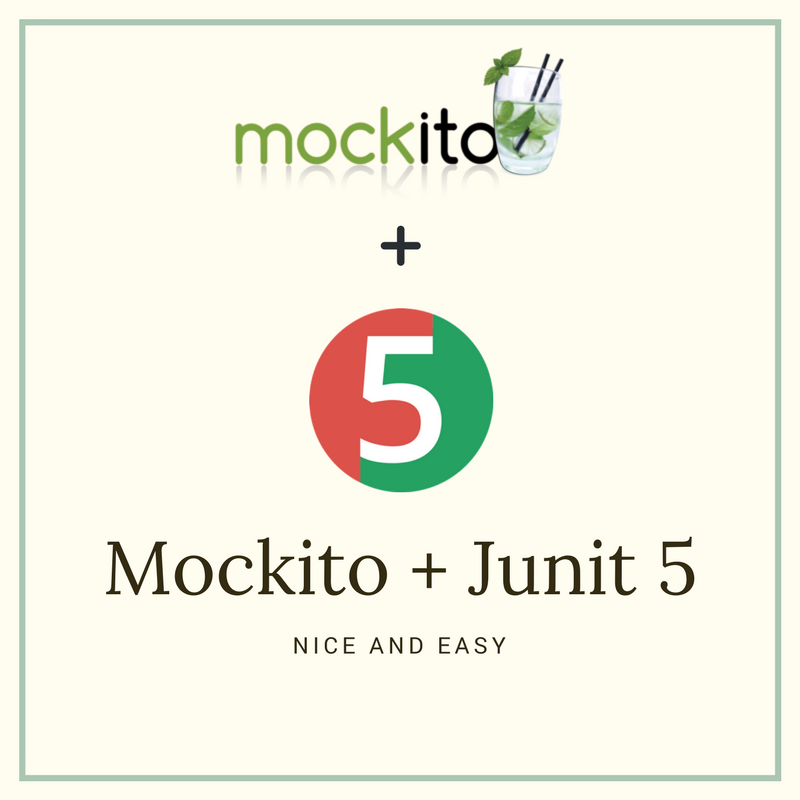
Since version 2.16.3 Mockito has official support for Junit5. Using Mockito with JUnit now is even easier than before. Previously I kept forgetting what rule I was supposed to use for injecting the mocks, and how I set the strictness again? Plus the extra field in each test class!
Mockito with JUnit 5 example
The newly introduced extension is not part of the mockito-core package. In order to use it, you will have to add a separate dependency:
<dependency> <groupId>org.mockito</groupId> <artifactId>mockito-junit-jupiter</artifactId> <version>${mockito.version}</version> <scope>test</scope> </dependency>
The extension will initialize all the fields annotated with @Mock. If you want to set the strictness of the stubs, use the MockitoSetting annotations. In the sample code, I set the strictness to STRTICT_STUBS although that is already the default.
@ExtendWith(MockitoExtension.class) @MockitoSettings(strictness = Strictness.STRICT_STUBS) class ChatEngineTest { @Mock private MessageSender messageSender; @Test public void shouldUseMessageSenderToSendMessage() { ChatEngine chatEngine = new ChatEngine(messageSender); String messageToSend = "Hello!"; chatEngine.say(messageToSend); when(messageSender.send(messageToSend)).thenReturn(OK); assertThat(messageSender.send(messageToSend)).isEqualTo(OK); } }
How does the extension work?
I previously spoke about the JUnit 5 extension model, and MockitoExtension is a great example of putting that into practice. It uses three extension points:
- TestInstancePostProcessor: to set the test instance.
- BeforeEachCallback: to create the Mockito session and initialize all the mocks.
- AfterEachCallback: to remove the previously created session.
Nice and easy.
Member discussion